– Register Bitbucket then import (or create a new one).
– Install SourceTree for controlling repository.
– Check out repo in SourceTree.
– Install node.js
– Install Git
– open cmd and go to target ‘directory’.
> npm install
> bower install
> npm install -g gulp
> npm start
– open another cmd and go to target ‘directory’.
> gulp watch
How to create a new element in jQuery:
var unreadMarker = $('<a>', { "class": "unread-marker" });
OR
var unreadMarker = $('<a class="unread-marker"></a>');
How to insert element:
a.after(b) ==> <a> <b>
a.insertAfer(b) ==> <b> <a>
a.append(b) ==> <a> xx yy b </a>
a.prepend(b) ==> <a> b xx yy </a>
function checkPhoneNumber(phone) {
var regexp = /^(?:(?:\(?(?:00|\+)([1-4]\d\d|[1-9]\d?)\)?)?[\-\.\ \\\/]?)?((?:\(?\d{1,}\)?[\-\.\ \\\/]?){0,})(?:[\-\.\ \\\/]?(?:#|ext\.?|extension|x)[\-\.\ \\\/]?(\d+))?$/i
if (regexp.test(phone) && phone.length > 0) {
return true;
} else {
return false;
}
}
Continue reading “Use regular expression to filter telephone number in JavaScript”
Add UIImagePickerControllerDelegate, UINavigationControllerDelegate
at top of the class.
Before viewDidLoad()
var picker = UIImagePickerController()
In viewDidLoad()
or any function that you want to use image picker
picker.delegate = self
Add some function for catch event
// What to do when the picker returns with a photo
//Use image that user pick from library
func imagePickerController(picker: UIImagePickerController, didFinishPickingMediaWithInfo info: [String : AnyObject]) {
let chosenImage = info[UIImagePickerControllerEditedImage] as! UIImage
dismissViewControllerAnimated(true, completion: nil)
addAvatar(chosenImage)
}
// What to do if the image picker cancels.
// some code for do anything when user cancel to import image
func imagePickerControllerDidCancel(picker: UIImagePickerController) {
dismissViewControllerAnimated(true, completion: {() in
})
}
Code for create UIScrollView (which named scrollView) and specify size of content (width and height) inside scrollView.
var scrollView = UIScrollView(frame: containerView.frame)
scrollView.contentSize = CGSize(width: 3000, height: 2000)
containerView.addSubview(scrollView)
// can be any view that is container of scrollView
Link of information about Data-binding Revolutions with Object.observe()
Material flat icons for web, Android, and iOS projects.
MZFormSheetPresentationController is an ios framework that provide a popup from UIViewController.
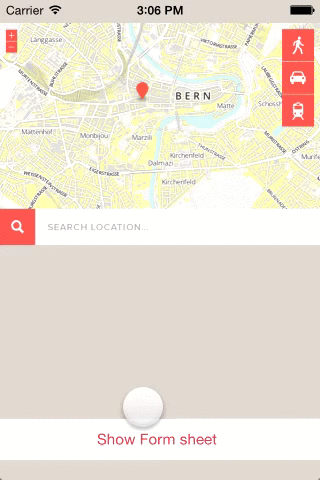
Step to integrate MZFormSheetPresentationController
1. add these 2 lines to podfile and compile
pod 'MZAppearance'
pod 'JGMethodSwizzler'
2. download .zip file from MZFormSheetPresentationController and extract
3. drag “MZFormSheetPresentationController” folder to your project
4. in your Bridging Header file, add these 2 lines
#import "MZFormSheetPresentationController.h"
#import "MZFormSheetPresentationControllerSegue.h"
5. create your new UIViewController .xib file, name it (Ex: PopupViewController.xib) and create your own popup layout.
6. Add these code in the UIViewController that you want to add your popup
class MainViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
var btn:UIButton = UIButton.buttonWithType(UIButtonType.InfoLight) as! UIButton
btn.addTarget(self, action: "btnInfoTouched", forControlEvents: UIControlEvents.TouchUpInside)
UIBarButtonItem(customView: btn)
self.navigationItem.rightBarButtonItem = UIBarButtonItem(customView: btn)
}
func btnInfoTouched(){
var infoViewController = UIViewController(nibName: "PopupViewController", bundle: nil)
var formSheetController = MZFormSheetPresentationController(contentViewController: infoViewController)
formSheetController.contentViewSize = CGSizeMake(280, 70)
formSheetController.contentViewControllerTransitionStyle = MZFormSheetPresentationTransitionStyle.StyleBounce
var tap:UITapGestureRecognizer = UITapGestureRecognizer(target: self, action: "dismissPopup")
formSheetController.view.addGestureRecognizer(tap)
// add tap gesture recognizer to detect tap and close popup
self.presentViewController(formSheetController, animated: true, completion: nil)
}
func dismissPopup() {
self.dismissViewControllerAnimated(true, completion: nil)
}
}
7. build and see the result
how to join array to string
var arrayList:[String]!
…..
var newString:String = “-“.join(arrayList)
&
how to explode string to array
var stringSentence:String!
…..
var newArray:[String] = stringSentence.componentsSeparatedByString(“-“)